All Things Typescript Newsletter - Issue #16 - Property Initialization in Classes
Good morning; I hope you had a fantastic weekend. This week we will cover property initialization in Typescript classes; on top of that, I have a few interesting articles you should read below to further your Typescript skills. I hope you will enjoy today’s issue, and if you have any questions or feedback, my DMs are open to you all.
I hope you have a great week ahead, and let’s keep on learning.
200+ Subscribers
I am excited that, as of today, we have over 200 subscribers to this newsletter. Please share with your friends and colleague and help me reach the next milestone for this newsletter and deliver awesome typescript content to you all.

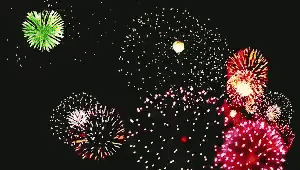
Property Initialization in Classes in Typescript
If you have worked with Angular and React (before hooks become the default way of writing React components), you may be familiar with classes in Typescript and JavaScript (in the case of React).
Here is an example of a Class in Typescript:
class Person {
firstName: string;
lastName: string;
}
Here, we have a class with two properties: firstName
and lastName
. As currently set up, we haven’t set values for both properties. We can set the values of the properties once we have created an instance of a class, as shown below:
const person = new Person()
person.firstName = "John"
person.lastName = "Doe"
It would be nice if we could set the values of the properties when initializing the class. Typescript provides a number of ways of achieving this. The first one is to set the value when declaring the property:
class Person {
firstName: string = "John";
lastName: string = "Doe";
}
The second option is to set values inside the constructor:
class Person {
firstName: string;
lastName: string;
constructor() {
this.firstName = "john"
this.lastName = "doe"
}
}
This second option has the same effect as the first option but does open the possibility of making deriving values for the property for instance we could now pass the firstName
and lastName
from the constructor and set them as properties.
class Person {
firstName: string;
lastName: string;
constructor(firstName: string, lastName: string) {
this.firstName = firstName;
this.lastName = lastName;
}
}
const person = new Person("John", "Doe")
But that does lead to a lot of boilerplate, so we can shorten the above code by using the constructor’s parameters to set class properties:
class Person {
constructor(public firstName: string, public lastName: string) {}
}
const person = new Person("John", "Doe")
The above code has the same effect as the previous one; as you can see, it’s more compact. You can replace public
with protected
, readonly
etc., based on the behaviors you expect from the class.
All Things Typescript Community
Last week, I was excited to announce a discord server where we, as members of the All Things Typescript community, can further our studies and get to know each other. This is a community for you people and where we can connect and learn.
One of the more exciting features of the community is going to be the weekly fireside chat that we will start having soon; we will be able to hold an hour of open audio/video discussion, where we can discuss any topic you think we need to discuss and get to know each other a little bit more.
If you want to join the community, here is a discord link. Feel free to ask any question, suggest future topics you might want to see me cove,r and get to network and know your fellow typescript learners.
Thank you.
Articles
Stronger JavaScript with Opaque Types
In Flow and TypeScript, types are transparent by default - if two types are structurally identical they are deemed to be compatible. For example, the following types are compatible:
Gentle Introduction To ESLint Rules
Have you ever wondered how your editor can know if you used var instead of let? or that you missed adding alt to the img tag? Yes, that is ESLint ladies and gentlemen. In this article, we’re going to talk about how this is possible and how you can do something similar!
Tidy TypeScript: Avoid traditional OOP patterns
This is the third article in a series of articles where I want to highlight ways on how to keep your TypeScript code neat and tidy. This series is heavily opinionated and you might find out things you don’t like. Don’t take it personally, it’s just an opinion.
Reduce maintenance effort with shared ESLint and Prettier configs
If you work on multiple projects, you might end up using the same ESLint and Prettier settings in each of them. Sure, using the same handy ESLint plugins and configurations is good for consistency, but you have to copy and paste your dependencies from your package.json, .eslintrc.js, and .
Documenting TypeScript, Finding the Right Tools for Your Project
TypeScript (TS) is becoming increasingly popular in the JavaScript (JS) ecosystem. Many popular libraries, like LibP2P, DiscordJS, Node-Postgres, and Selenium Driver, have already started rewriting their JavaScript codebase in TypeScript, with many others following their lead.
What’s New in ES2022? 4 Latest JavaScript Features
The new ES12 specs are finally released. Specs? JavaScript is indeed not an open-source language. It’s a language written following the ECMAScript standard specifications. The TC39 committee is in charge of discussing and approving new features. Who are they?
Effective Do’s and Don’ts for Your Next Code Review
Being a great coder doesn’t mean only writing clean code. It also means knowing how to read other people’s code, understand it, and give useful feedback on how best to iterate on it.
Why we should verify HTTP response bodies, and why we should use zod for this
Okay, it’s time to take a look at zod. The first time I encountered zod was because it was a recommendation on the GitHub dashboard, the second time was the nomination for the OS awards for the “Productivity Booster” category.
Tweets

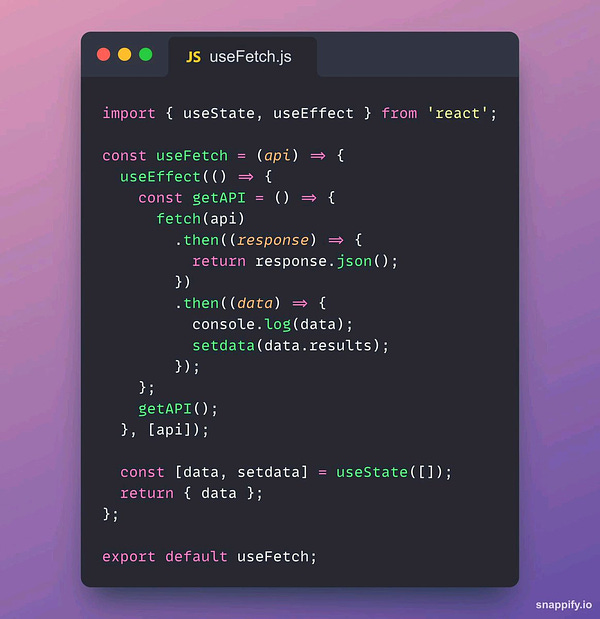

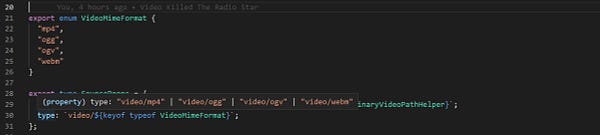