The Tuple Type in Typescript - All Things Typescript Newsletter - Issue #19
Learn more about the Tuple Type in Typescript
Good Afternoon; I hope you had a fantastic weekend. First, I am excited about a few things: First, we will now have two issues per week, with a mid-week scoop short form article containing tips and tricks you can use to understand and get the most out of Typescript. Please subscribe to not miss out on this week’s scoop on Interfaces vs. Types.
Secondly, my GitHub sponsor profile is live. If you are looking to support my work on content creation, open source contribution, and mentoring, please do to enable me to be able to dedicate more to doing what I love.
And lastly, I started doing live streaming solving various challenges on YouTube; and I did my first live stream on Monday. Tonight, I have another live stream where I will get to set up tests for my NPM Imported Package Links VS Code Extension using jest.

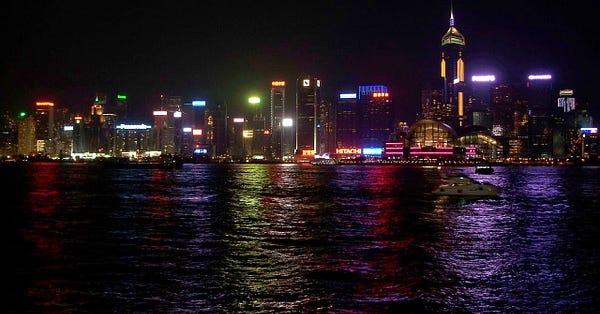
The Tuple Type in Typescript
A tuple type is an array with a predefined length and predefined types in each index position in the array. The types inside this array do not have to be the same, they could be a mixture of different types. A normal array can contain anywhere from zero to an unknown number of elements, and the order is not important.
This is where a Tuple differentiates itself from an array. In a tuple, the type of each element, the length of the array, and the order in which the elements are ordered in the array are essential. I.e., it should always return an array of length 2, with the first element being a string and the second element being a number.
To define a Tuple type, we use syntax similar to Javascript array syntax, but instead of specifying the values, we specify the type in each index location, as shown below.
type PersonNameAge = [string, number];
In the example above, we are defining a Tuple type PersonaNameAge
, as an array of length two, with the first element being a string for person's name and the next element being a number for a persons' age.
We can then go ahead and use the above tuple as follows:
const personNameAge: PersonNameAge = ["John Doe", 25] // this doesn't throw an error
If we don't provide enough elements matching the length of fields defined in the PersonNameAge
tuple, then Typescript will throw the following error:
const personNameAge: PersonNameAge = []
Error: Type '[]' is not assignable to type 'PersonNameAge'. Source has 0 element(s) but target requires 2.
Typescript will also throw an error if you provide more elements:
const personNameAge: PersonNameAge = ["John Doe",25, true]
ERROR: Type '[string, number, number]' is not assignable to type 'PersonNameAge'. Source has 3 element(s) but target allows only 2.
And if we specified the types not matching the types specified in their index location, Typescript will throw the following error i.e. let’s say Typescript reversed the name and age, as shown below:
const personaNameAge: PersonNameAge = [25,"John Doe"]
ERROR: Type 'string' is not assignable to type 'number'.(2322)
Why Tuple
Tuples have several benefits, the first one being able to return more than one value from a function. Take for instance the following function:
function doSomething(): [string, number] {
// do something
}
It can return two values, a string and a number, which the caller can assign to variables. This leads to the second benefit, being able to destructure them easily to a variable name of choice i.e. being able to assign the return values of the tuple directly to their variables as shown below.
const [str, nmb] = doSomething();
If you returned an object instead of a tuple, destructing takes an extra step of needing to rename the field, especially if there is a variable name collision. You can also ignore the return type of Tuple by using an underscore (_)
character if you wanted to access the value of a variable that is in a much higher index position.
const [_, nmb] = doSomething();
Announcements 📢
Announcing TypeScript 4.8 RC - TypeScript
Today we’re excited to announce our Release Candidate (RC) of TypeScript 4.8. Between now and the stable release of TypeScript 4.8, we expect no further changes apart from critical bug fixes.
Articles
Create a Static Class in Typescript
You can't have static
classes in Typescript
out of the box, but there are several approaches to make a class that cannot be instantiated. This article is about them.
An Introduction to Conditional Types in TypeScript
Conditional types in TypeScript give us the ability to define certain types based on logic, just like we do in other aspects of our code. They are a useful tool in defining types in TypeScript.
Extending Global Event System with Typescript Generics
In the first part we have gone through the creation of the initial Event System. It allowed tracking events happening anywhere in the code and trigger handlers for these events.
How to build rich, accessible JavaScript interfaces
So you’re a JavaScript whiz who can build pretty much anything — but can everyone use it? In this article, we’ll look at some ways to make rich, accessible JavaScript interfaces. We’ll illustrate our strategies by building a simple Sudoku puzzle that works for everyone, even those who are blind or partially sighted.
How to extend the Express Request object in TypeScript
The Request
object is used by Express to provide data about the HTTP request to the controllers of a Node.js server. Therefore, all data from the request available at the application layer depends on this object.
Tweets



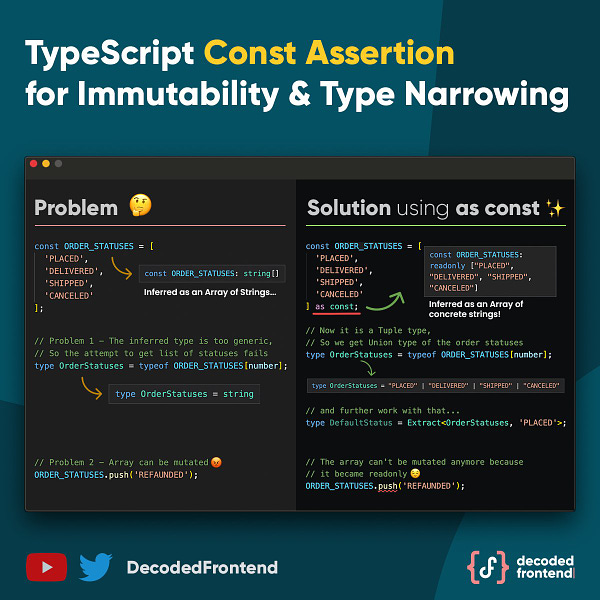
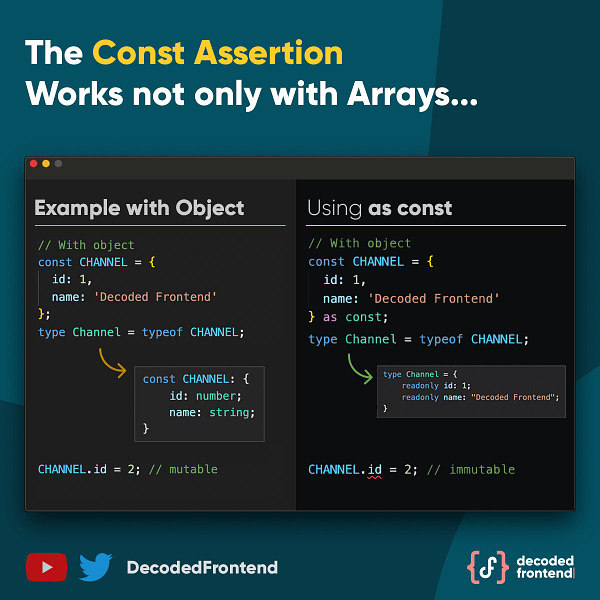
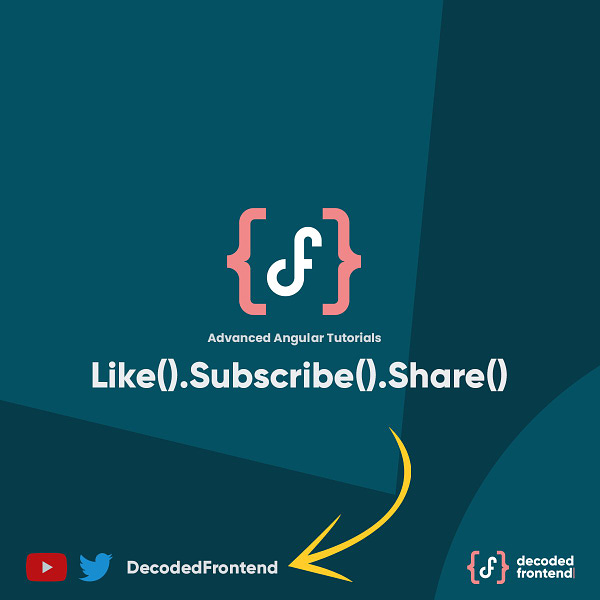


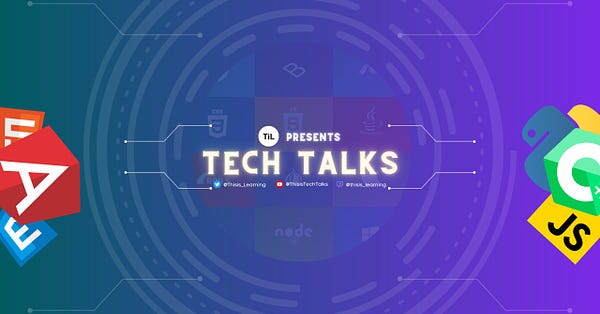
